1. このページの目的
User Timing Level 2 の mark()
メソッドを試す。
2. Demo
a. 本ページで実行するソースコード(抜粋)
document.querySelector('#btn1').addEventListener('click', function() {
// 計測開始
performance.mark("startTask1");
// 何らかの重い処理を実行する
let primes = eratosthenes(50000000);
// 計測終了
performance.mark("endTask1");
// measure() を実行する
performance.measure('Task1', 'startTask1', 'endTask1');
console.log(performance.getEntriesByName('Task1', 'measure'));
// PerformanceEntry を取得して出力する
const entries = performance.getEntriesByType("mark");
for (const entry of entries) {
console.table(entry.toJSON());
}
});
- 最後の for の部分はなくてもよい。
b. 実行するボタン
DevTools の Console パネルを開いてから、ボタンを押して下さい。
3. 結果のスクリーンショット
DevTools の Console パネルに表示された内容のスクリーンショットです。
measure()
の戻り値(PerformanceMeasure オブジェクト)
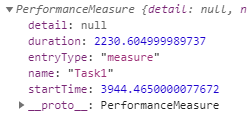
- 掛かった時間は、
duration
にセットされている。
"startTask1" という名前をつけた PerformanceMark オブジェクト
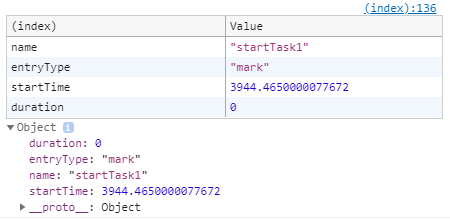
"endTask1" という名前をつけた PerformanceMark オブジェクト
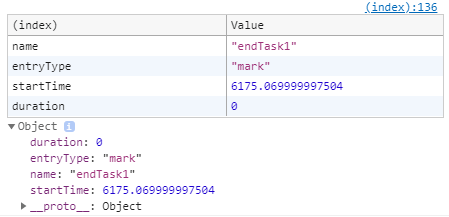
4. 参考情報
(1) window.performance
window.performance
は、Performance オブジェクトである。- PerformanceObserver インターフェースとは別ものである。
(2) PerformanceEntry interface
WebIDL
[Exposed=(Window,Worker)]
interface PerformanceEntry {
readonly attribute DOMString name;
readonly attribute DOMString entryType;
readonly attribute DOMHighResTimeStamp startTime;
readonly attribute DOMHighResTimeStamp duration;
[Default] object toJSON();
};
(3) Performance interface
Performance
interface は、PerformanceEntry
interface を拡張している。
WebIDL
partial interface Performance {
void mark(DOMString markName);
void clearMarks(optional DOMString markName);
void measure(DOMString measureName, optional DOMString startMark, optional DOMString endMark);
void clearMeasures(optional DOMString measureName);
};
measure()
メソッドの引数に注意する。
(4) ブラウザがサポートしている entryType を判定するコード
1. Introduction | EXAMPLE 1 | Performance Timeline Level 2 に載っているコード
// Know when the entry types we would like to use are not supported.
function detectSupport(entryTypes) {
for (const entryType of entryTypes) {
if (!PerformanceObserver.supportedEntryTypes.includes(entryType)) {
// Indicate to client-side analytics that |entryType| is not supported.
}
}
}
detectSupport(["resource", "mark", "measure"]);
(5) window.performance
と PerformanceObserver
インターフェースの関係
window.performance
はPerformance
インターフェースを実装したオブジェクトである。PerformanceObserver
インターフェースではない。new PerformanceObserver()
で生成したオブジェクトは、対象となる entryType を持ったオブジェクト(window.performance
も含む)のパフォーマンス計測動作を監視することができる。- 例えば、本ページのコードに以下のコードを追加すると、
window.performance
で発生した動作も監視することができる。
new PerformanceObserver(e => {
for (const o of e.getEntries())
console.log(o)
}).observe({ entryTypes: ["mark", "measure"] });